Algorithm design is the process of creating a step-by-step procedure for solving a problem or achieving a specific task. This process involves understanding the problem or task at hand, identifying the inputs and outputs, and developing a logical sequence of steps to produce the desired result. Algorithm design can be applied to a wide range of fields, including computer science, mathematics, and engineering. Good algorithm design is characterized by being efficient, correct, and easy to understand and implement.
There are several key techniques used in algorithm design, including:
- Brute force: This involves trying all possible solutions to a problem, and is typically not very efficient.
- Divide and conquer: This involves breaking a problem down into smaller subproblems that can be solved independently, and then combining the solutions to solve the original problem.
- Dynamic programming: This involves breaking a problem down into smaller subproblems and storing the solutions to these subproblems in a table, so that they can be reused.
- Greedy algorithm: This involves making the locally optimal choice at each step, with the hope of reaching a global optimal solution.
- Backtracking: This involves trying different solutions, and undoing the solutions that do not lead to a correct answer.
- Randomized algorithm: This involves using randomness in the algorithm, either to make decisions or to generate solutions.
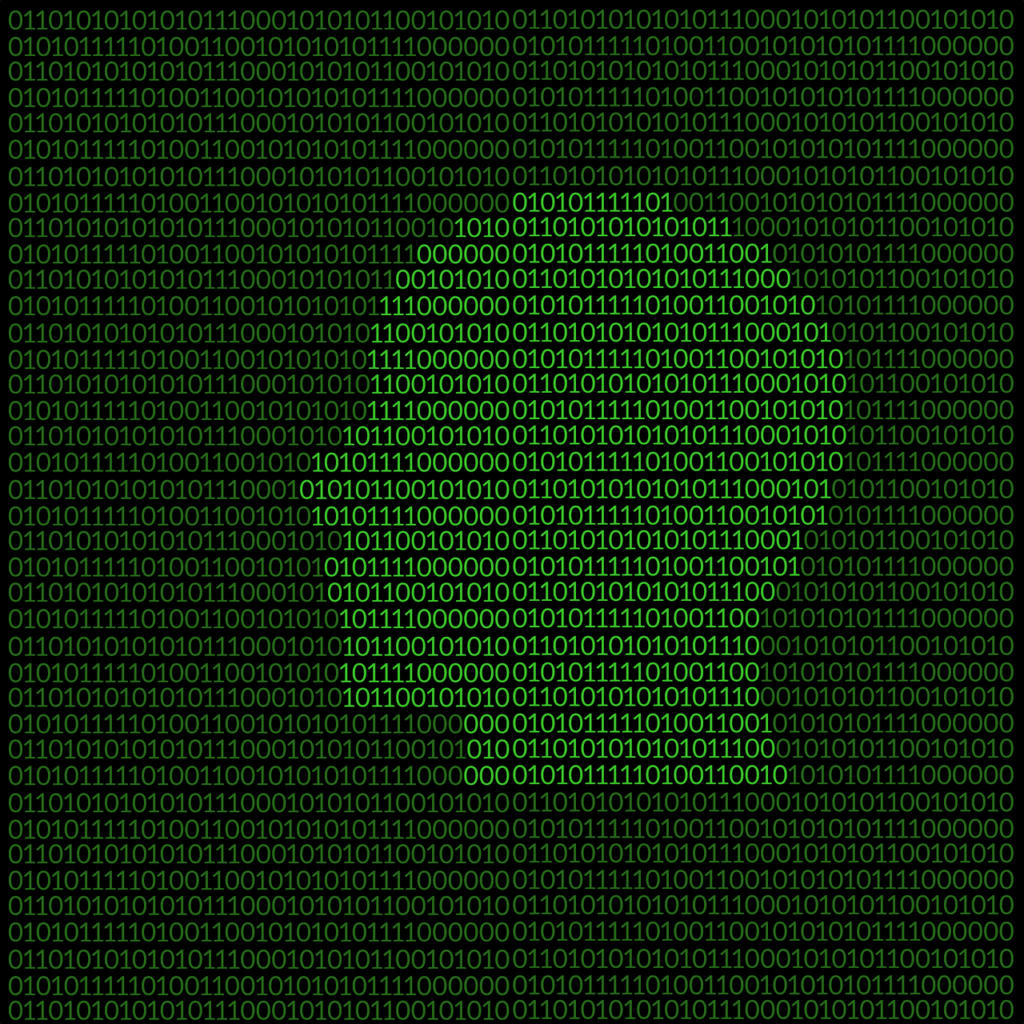
It’s important to note that there is no one-size-fits-all algorithm design and the best solution depends on the problem, you need to select the technique that best suits the problem at hand and will be the most efficient and effective.
Another important aspect of algorithm design is analyzing the time and space complexity of an algorithm. Time complexity is a measure of how long an algorithm takes to run, based on the size of the input. Space complexity is a measure of how much memory an algorithm uses, also based on the size of the input.
Time and space complexity of an algorithm
Time complexity of an algorithm is a measure of how long the algorithm takes to run, based on the size of the input. It is typically expressed using big O notation, which describes the worst-case scenario for the algorithm’s running time.
There are several common time complexity classes, including:
- O(1), also known as constant time, is an algorithm that takes the same amount of time to run regardless of the size of the input.
- O(log n) is an algorithm that takes a logarithmic amount of time to run, and is generally considered very efficient.
- O(n) is an algorithm that takes linear time to run, and is generally considered to be of moderate efficiency.
- O(n log n) is an algorithm that takes a logarithmic amount of time to run for each item in the input, and is generally considered to be efficient.
- O(n^2) is an algorithm that takes a square of time to run, and is generally considered to be of moderate efficiency.
- O(2^n) and O(n!) are algorithms that takes an exponential time to run, and are generally considered to be very inefficient.
Space complexity of an algorithm is a measure of how much memory the algorithm uses, based on the size of the input. Like time complexity, it is also typically expressed using big O notation. Some common space complexity classes are:
- O(1), also known as constant space, is an algorithm that uses a fixed amount of memory regardless of the size of the input.
- O(n) is an algorithm that uses linear space, proportional to the size of the input.
- O(n log n) is an algorithm that uses a logarithmic amount of space for each item in the input.
- O(n^2) is an algorithm that uses a square of space, proportional to the square of the size of the input.
- O(2^n) and O(n!) are algorithms that uses an exponential space, which grow very quickly with the size of the input and are generally considered to be very inefficient.
It’s important to note that both time and space complexity are closely related, and often a trade-off exists between them, an algorithm that uses less space might be slower, or an algorithm that uses less time might use more space.
It’s important to strive for an algorithm that has the best possible time and space complexity, since it can greatly impact the performance of the program.
In addition to the above, there are more sophisticated methodologies that can be used to analyze the performance of an algorithm like the Amortized analysis, Randomized analysis, Approximation Algorithms and more.
The importance of time and space complexity analysis
The importance of time and space complexity analysis in algorithm design is that it allows us to understand the efficiency and scalability of an algorithm. By understanding the time and space complexity of an algorithm, we can make informed decisions about which algorithm to use for a given problem, and how to optimize the algorithm for better performance.
For example, if we have an algorithm with a time complexity of O(n^2) and we are working with a large dataset, it may take an unacceptably long time to run. In this case, we may want to consider an alternative algorithm with a better time complexity, such as O(n log n) or O(n).
In terms of space complexity, it is important because in some cases, the amount of memory required for an algorithm can be a limiting factor. For example, if we are working with a large dataset and our algorithm has a space complexity of O(n^2), it might require an excessive amount of memory and cause the system to crash.
Another important aspect of algorithm design is testing and verification. Once an algorithm has been designed, it should be thoroughly tested to ensure that it produces the correct results for a wide range of inputs. This can involve creating test cases that cover edge cases and corner cases, as well as more typical inputs. It’s important to consider the performance of the algorithm, and it should be tested with inputs of varying sizes to ensure that it scales well.
Additionally, it’s important to consider the use of formal methods for verification, like mathematical proof and model checking, which can provide a higher level of assurance that the algorithm is correct and complete.
Lastly, it’s important to consider the maintainability and readability of the algorithm. The algorithm should be well-documented, with clear variable and function names, and it should be easy to understand and modify. This is important for long-term projects, as the code may need to be modified and maintained by others.
Important aspect of algorithm design is testing and verification
Testing and verification are important steps in the algorithm design process, as they help to ensure that the algorithm produces the correct results for a wide range of inputs.
Testing involves creating a set of test cases that cover different scenarios, such as edge cases, corner cases, and typical inputs. These test cases are used to evaluate the performance and correctness of the algorithm. It’s important to consider the performance of the algorithm and it should be tested with inputs of varying sizes to ensure that it scales well.
Verification, on the other hand, is the process of formally proving that the algorithm is correct and complete. One way to do this is through mathematical proof, where we can use mathematical notation and logic to prove that the algorithm will always produce the correct result. Another way to verify an algorithm is through model checking, where we use a formal model of the algorithm and a set of properties that the algorithm should satisfy, and then use automated tools to check if the algorithm meets those properties.
By testing and verifying an algorithm, we can gain a high level of assurance that the algorithm is correct and complete, and that it will work as expected when applied to real-world problems. This can also help to identify and fix any bugs or errors that may exist in the algorithm.
Formal methods for verification
Formal methods for verification are mathematical techniques used to formally prove the correctness and completeness of an algorithm. They provide a higher level of assurance that the algorithm is correct and will produce the expected results for any input.
Some common formal methods for verification include:
- Mathematical proof: This involves using mathematical notation and logic to prove that an algorithm will always produce the correct result for a given input. This can be done using proof by induction, contradiction, or contrapositive, among other methods.
- Model checking: This involves using a formal model of the algorithm and a set of properties that the algorithm should satisfy, and then using automated tools to check if the algorithm meets those properties.
- Hoare Logic: Hoare Logic is a method of reasoning about computer programs that uses formal rules to infer pre- and postconditions of a program fragment. It provides a way to reason about the correctness of a program.
- Temporal Logic: Temporal Logic is a formalism that allows to express properties of dynamic systems, including algorithms. It provides a way to reason about the behavior of the system over time.
- Formal verification by testing: This involves using formal methods to generate test cases that can be used to check if the algorithm meets certain properties.
These formal methods can be used to prove the correctness of algorithms and to verify that they work as intended. They can also help to identify and fix any bugs or errors that may exist in the algorithm, making it more reliable and robust.
It’s important to note that formal verification is a complex and time-consuming process, and it might not be feasible to use formal methods for all algorithms, but it’s a good practice to consider them when working on critical systems, where the cost of failure is high.
Importance of Algorithm design
Algorithm design is an important field of computer science and problem-solving, as it allows us to develop effective and efficient solutions for a wide range of problems. The importance of algorithm design can be summarized in several points:
- Problem-solving: Algorithm design provides a systematic and logical approach to solving problems, which can be applied to a wide range of fields, including computer science, mathematics, and engineering.
- Efficiency: Good algorithm design leads to efficient solutions that can handle large inputs and scale well. This is particularly important in fields such as big data and machine learning, where the amount of data to be processed can be extremely large.
- Correctness: Algorithm design helps to ensure that the solution is correct, by providing a step-by-step procedure that produces the desired results. This can be verified through testing and formal verification methods.
- Maintainability: Algorithm design helps to create solutions that are easy to understand, modify, and maintain over time, which is important for long-term projects and collaborations.
- Innovation: Algorithm design is a creative process that can lead to new and innovative solutions for problems.
In summary, the importance of algorithm design lies in its ability to provide efficient, correct, and maintainable solutions for a wide range of problems, and to foster innovation and creativity in problem-solving.
Type of Algorithms
Algorithms can be categorized into several types based on their purpose, structure, and design. Here are some of the general types of algorithms:
- Sorting algorithms: These algorithms are designed to arrange data in a particular order, such as ascending or descending. Examples of sorting algorithms include Bubble Sort, Quick Sort, and Merge Sort.
- Searching algorithms: These algorithms are used to find a specific piece of data within a collection of data. Examples of searching algorithms include Linear Search, Binary Search, and Hashing.
- Graph algorithms: These algorithms are used to solve problems related to graphs, such as finding the shortest path between two points or determining if a graph is connected. Examples of graph algorithms include Breadth-First Search, Depth-First Search, and Dijkstra’s Algorithm.
- Divide and conquer algorithms: These algorithms break down a problem into smaller sub-problems, solve each sub-problem separately, and then combine the solutions to solve the original problem. Examples of divide and conquer algorithms include Binary Search, Merge Sort, and Quick Sort.
- Dynamic programming algorithms: These algorithms use previously computed solutions to solve complex problems more efficiently. Examples of dynamic programming algorithms include Fibonacci Series, Knapsack Problem, and Longest Common Subsequence.
- Brute-force algorithms: These algorithms solve a problem by trying every possible solution until a solution is found. Examples of brute-force algorithms include the Traveling Salesman Problem and the Subset Sum Problem.
- Greedy algorithms: These algorithms make locally optimal choices at each step with the hope of finding a global optimum. Examples of greedy algorithms include Dijkstra’s Algorithm and Kruskal’s Algorithm.
These are just a few examples of the general types of algorithms, and there are many other specialized algorithms designed to solve specific types of problems.
Summary
Algorithm design is the process of creating a step-by-step procedure for solving a problem or achieving a specific task. It involves understanding the problem or task at hand, identifying the inputs and outputs, and developing a logical sequence of steps to produce the desired result. Algorithm design can be applied to a wide range of fields, including computer science, mathematics, and engineering. Good algorithm design is characterized by being efficient, correct, and easy to understand and implement.
There are several key techniques used in algorithm design, including brute force, divide and conquer, dynamic programming, greedy algorithm, backtracking, and randomized algorithm. Analyzing the time and space complexity of an algorithm is also an important aspect of algorithm design, as it allows us to understand the efficiency and scalability of an algorithm. Time complexity is a measure of how long an algorithm takes to run, based on the size of the input, and space complexity is a measure of how much memory an algorithm uses, also based on the size of the input.
Testing and verification are also important steps in the algorithm design process, as they help to ensure that the algorithm produces the correct results for a wide range of inputs. Verification, specifically, is the process of formally proving that the algorithm is correct and complete, using formal methods like mathematical proof, model checking, Hoare Logic, Temporal Logic, and formal verification by testing.
In summary, algorithm design is a multi-faceted process that involves understanding the problem, designing a solution, analyzing the performance, testing and validating the results, and ensuring that the solution is maintainable and readable. The importance of algorithm design lies in its ability to provide efficient, correct, and maintainable solutions for a wide range of problems, and to foster innovation and creativity in problem-solving.
[…] are several algorithms for finding a spanning tree of a graph, including Prim’s algorithm, Kruskal’s algorithm, and […]
[…] not hold. Alternatively, we can use the Deadlock Avoidance solution by implementing the Banker’s algorithm, which ensures that the system always remains in a safe […]
[…] algorithm is similar to Dijkstra’s algorithm, but it works by processing the graph in “buckets” of […]
[…] learning algorithms are machine learning algorithms that learn to make predictions by learning patterns from labeled data. There are several types of […]
[…] Intelligence (AI) algorithms are at the heart of the AI revolution that is transforming the way we live and work. AI algorithms […]